Guest Join SDK
Cobrowse includes the ability for agents to optionally admit unauthenticated "guests" into sessions as additional participants to view the visitor's browser.
Glance supplies a default guest join experience for each group. To see what this looks like, navigate to the following URL, substituting your group ID for the parameter at the end:
https://www.glance.net/guest/?groupid=<groupid>
In addition to the out-of-the-box flow, Glance also offers the Guest Join SDK, which allows you to customize the experience. If you choose this option, you must supply the UI entirely.
Complete the following steps to implement the Guest Join SDK:
1. Reference the SDK File
The Guest Join SDK can be utilized by linking to the following URL:
- Staging = glance.net/script/
<groupid>
/staging/Guest - Production = glance.net/script/
<groupid>
/production/Guest
NOTE:
groupid
in the above URLs must be replaced with your Group ID.
Below is an example script source tag that could be added your Guest Join page:
<script src="https://www.glance.net/script/12345/production/Guest"></script>
Note: In the example above, 12345 is used as a Group ID, you would need to update this value with your Group ID.
2. Construct the UI
At a basic level, the act of admitting a guest to a Cobrowse session involves:
- Connecting to the Glance service from the guest's device.
- Receiving a code from the Glance service (i.e., the guestcode).
- Communicating the guestcode to the agent so the agent can use it to admit the guest.
Generally, the above flow is accomplished via a web interface made available to the guest, which involves building:
- A webpage where the guest can optionally enter their name and click a button to generate a 6-digit code (the guestcode).
- A page that displays the guestcode once generated.
- Some error handling/messaging.
For example, you could build a page similar to the default guest join experience that looks like this:
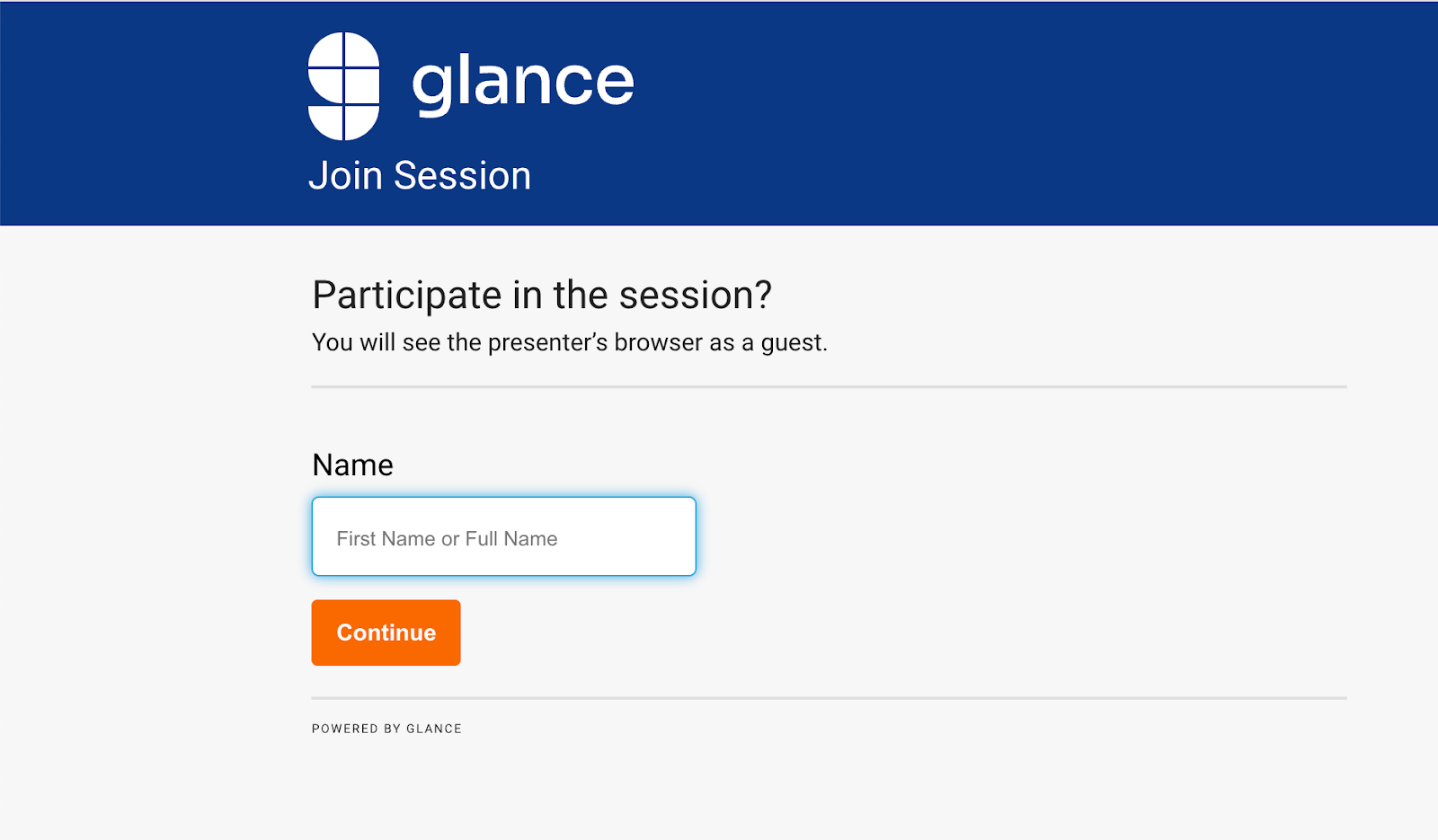
It contains one form field and one button. When the guest clicks Continue, your page will run code similar to the following, leveraging the Glance SDK in various places:
async function getJoinCode() {
// define the guest's name, or use the generic "Guest" if the field is blank
var guestName = document.querySelector("#GuestName").value
? document.querySelector("#GuestName").value
: "Guest";
// Show or hide UI as appropriate, for example:
$("#headermessagetext").hide();
$("#guestname").hide();
$("#waitingtext").show();
$("#joincode").show();
let connector = new GLANCE.Guest.Connector({
groupid: groupid, // Make sure to replace with your unique group id
ws: "www.glance.net",
});
connector.addEventListener("timeout", () => {
// Perform functions/adjust UI in the event that the agent fails to admit the guest before the code times out
});
connector.addEventListener("error", () => {
// Perform functions/adjust UI in the event of an error
});
let guestcode = await connector.connect(guestName);
if (!guestcode.ok) {
// Perform functions/adjust UI in the event of an error
} else {
// guestcode.code contains the code to be displayed to the user. Adjust the UI and display it to the user.
// guestcode.timeout indicates the number of seconds until the code will timeout.
// You may optionally use this to extend the timeout, possibly in response to a user prompt if desired.
}
}
Note: The code example above is for illustrative purposes only. It will need to be adjusted based on the exact design of your page and cannot be used as is.
The resulting page could look something like this:
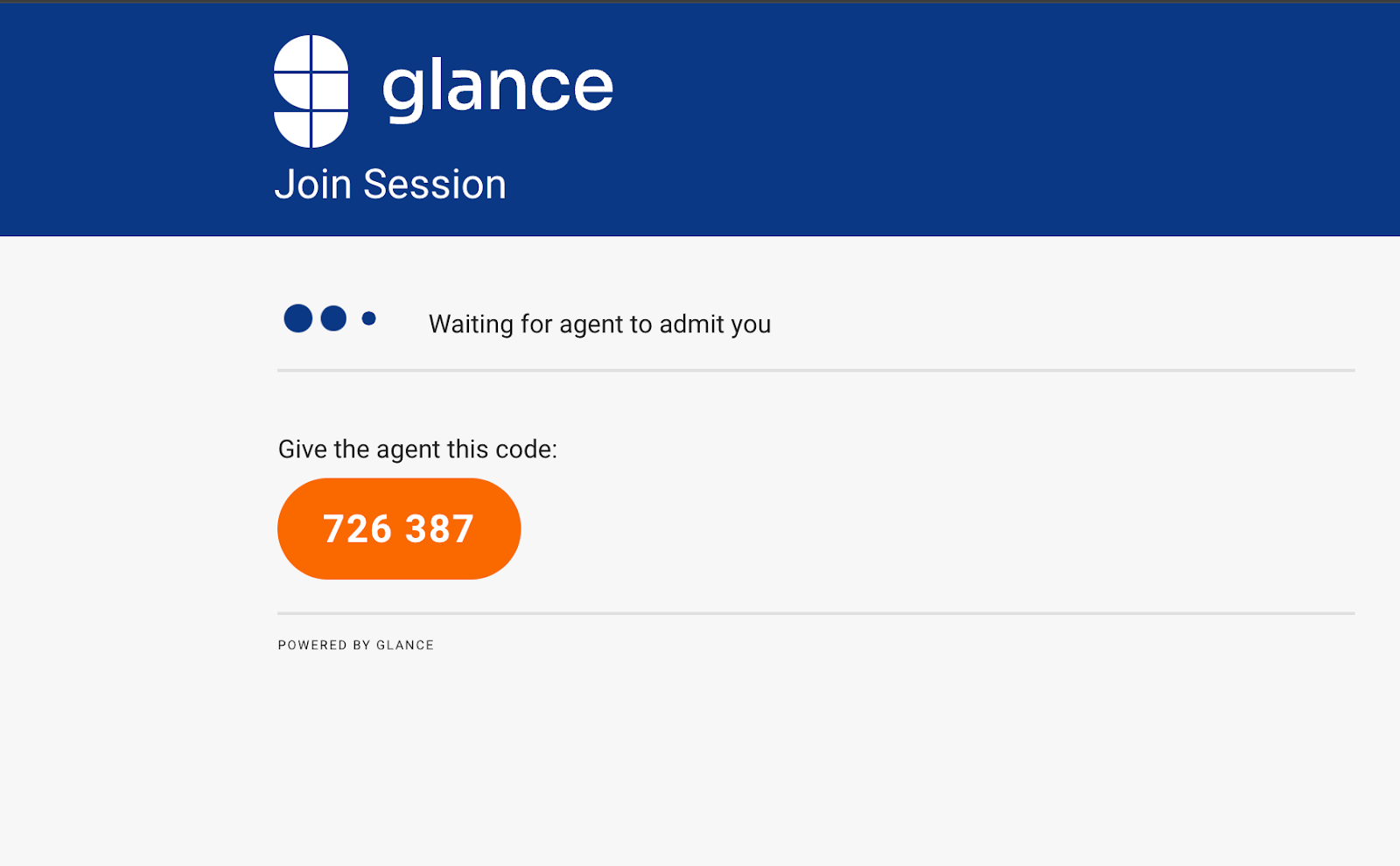
When the agent admits the guest successfully, the guest's browser will automatically be redirected to the Guest Viewer page (hosted on glance.net) so this event does not need to be handled explicitly.
After the session, the guest is redirected to a URL that can be specified in the Account Management area of glance.net.
Refer to the Glance Guest API Reference Document for a breakdown of the events, parameters, and additional functionality available.