Quickstart
1. Install the SDK
The Mobile SDK for iOS is available for installation via Cocoapods or Manual integration.
Cocoapods
Add Glance_iOS framework into your Podfile:
pod 'Glance_iOS'
Run pod_install in your terminal:
pod install
Manual
Download Glance_iOS from here.
Drag the xcframework to your XCode project and select Copy items if needed.
Make sure it was added to Frameworks and Libraries section in the General tab from your target Settings, don't forget to select the embed option Embed & Sign.
2. Initialize the SDK
On application startup, initialize the SDK with the following lines:
import Glance_iOS
...
GlanceVisitor.initVisitor(GLANCE_GROUP_ID, token: "", name: "", email: "", phone: "")
#import <Glance_iOS/Glance_iOS.h>
...
[GlanceVisitor init:GLANCE_GROUP_ID token:@"" name:@"" email:@"" phone:@""];
import Glance_iOS
...
func application(_ application: UIApplication, didFinishLaunchingWithOptions launchOptions: [UIApplicationLaunchOptionsKey: Any]?) -> Bool
{
...
// Configure Glance Visitor SDK
GlanceVisitor.initVisitor(GLANCE_GROUP_ID, token: "", name: "", email: "", phone: "")
return true
}
#import <Glance_iOS/Glance_iOS.h>
...
- (BOOL)application:(UIApplication *)application didFinishLaunchingWithOptions:(NSDictionary *)launchOptions {
...
// Configure Glance Visitor SDK
[GlanceVisitor init:GLANCE_GROUP_ID token:@"" name:@"" email:@"" phone:@""];
return YES;
}
3. Start sharing your screen
To start sharing your screen, call the startSession() method like this:
GlanceVisitor.startSession()
[GlanceVisitor startSession];
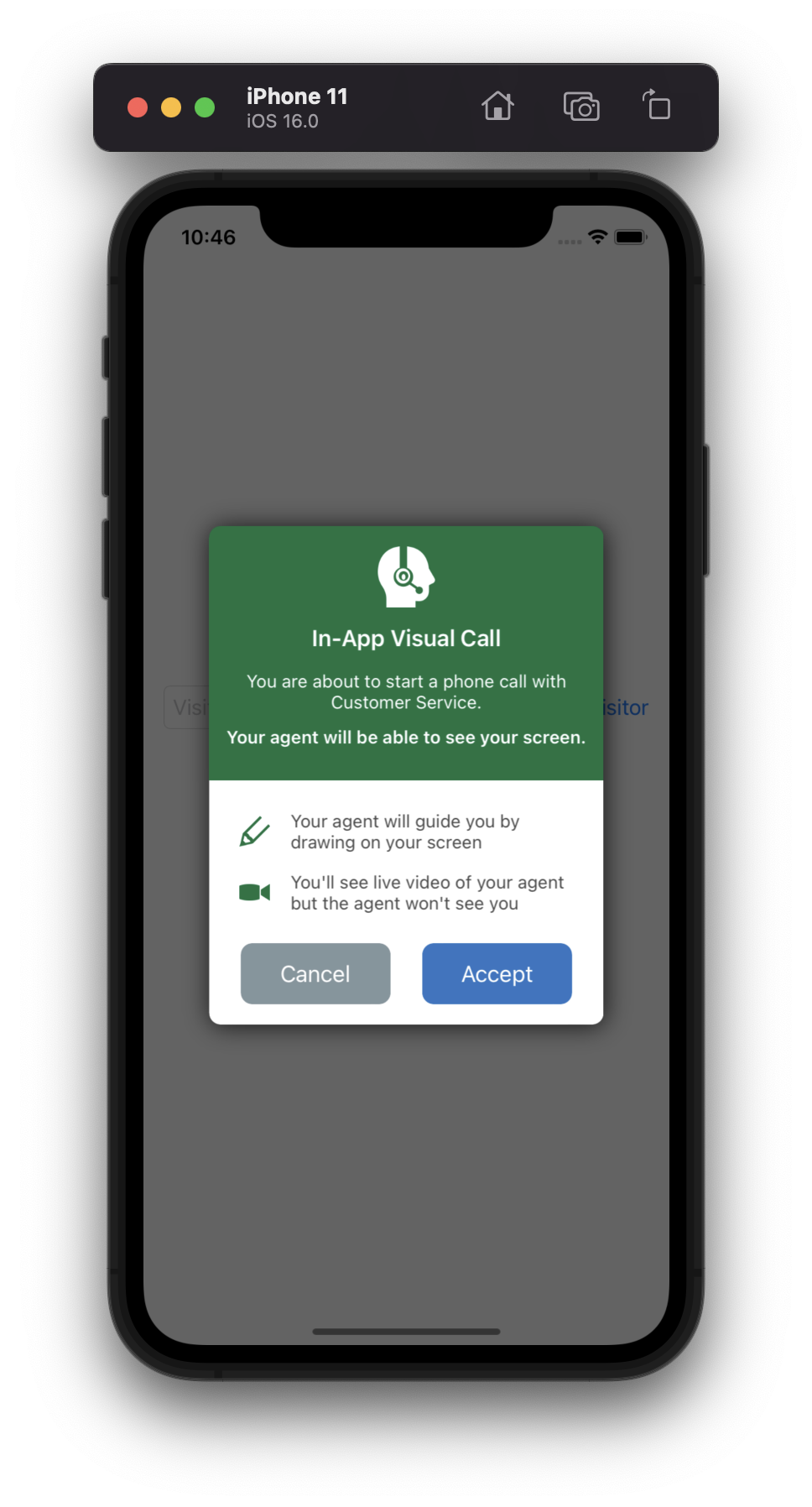
Then you'll see a code like the screen below:
Copy the code from the screen and send to your Agent, once they accept the call, you will be sharing your screen.
You can also define a Session Code when calling startSession()
GlanceVisitor.startSession("22333")
[GlanceVisitor startSession:@"22333"];
4. Testing the Agent
To confirm the integration is working, start the session and note the session key.
Now, to simulate the Agent, go to: https://www.glance.net/agentjoin/AgentJoin.aspx.
If not logged in already, the agent will be asked to login, ask credentials to Glance if you still don't have them:
Now, enter the Session Code and tap Join Session:
After that, you'll be able to see your mobile device screen.
5. Receiving events
To receive events from the SDK, you need to implement the protocol GlanceVisitorDelegate like below:
class MyGlanceVisitorDelegate: GlanceVisitorDelegate {
func glanceVisitorEvent(_ event: GlanceEvent!) {
DispatchQueue.main.async {
switch event.code {
case EventSessionEnded:
// Successfully connected to session
// Get generated session key
break
case EventConnectedToSession:
// Couldn't start session
// Error message can be found in event.message
break
case EventStartSessionFailed:
// Session ended
break
default:
// It is recommended that you at log all other events to help debug during development
// Best practice is to log all other events of type EventAssertFail, EventError, or EventWarning
break
}
}
}
}
@protocol GlanceVisitorDelegate
-(void)glanceVisitorEvent:(GlanceEvent*)event;
@end
-(void)glanceVisitorEvent:(GlanceEvent*)event{
switch (event.code)
case EventConnectedToSession:
// Successfully connected to session
// Get generated session key
break;
case EventStartSessionFailed:
// Couldn't start session
// Error message can be found in event.message
break;
case EventSessionEnded:
// Session ended
break;
default:
// It is recommended that you at log all other events to help debug during development
// Best practice is to log all other events of type EventAssertFail, EventError, or EventWarning
break;
}
}
Finally, we need to add our new delegate to the SDK, otherwise our delegate will not receive any events:
GlanceVisitor.add(glanceVisitorDelegate)
[GlanceVisitor addDelegate: self];
6. Masking
Views with personal private information or banking information should be hidden from the agent. You can mask a view like this:
GlanceVisitor.addMaskedView(self.creditCardNumberTextField)
[GlanceVisitor addMaskedView: self.creditCardNumberTextField];
You can remove a mask with another call:
GlanceVisitor.removeMaskedView(self.creditCardNumberTextField)
[GlanceVisitor removeMaskedView: self.creditCardNumberTextField];
Congratulations! You have the basic screen share flow implemented.